Wiki
Clone wikimodflow_utilities / Home
Getting Started
This page shows how to use and verify the code under your system.
Reading head output
To read the output head file use the readModflowArrays
function:
#!matlab H = readModflowArrays(['Example_files/gvmod.hds']);
The file gvmod.hds contains the head distribution of a single layer aquifer. The simulation is transient state with 119 stress periods.
The output of the command is a matrix where each row containts the head distribution for one layer of one time step of one stress period. Each row is actually a struct variable with the following fields:
-
KST : the time step number
-
KPRE : the stress period number
-
PERTIM : the time in the current stress period
-
TOTIM : the total elapsed time
-
DESC : a description of the array
-
NCOL : the number of columns in the array
-
NROW : the number of rows in the array
-
ILAY : the layer number
-
data : A matrix [NROWxNCOL].
To plot the head distribution in matlab is as easy as
#!matlab surf(H(1,1).data)

However as you can see the figure is not very informative. The reason is that the no-flow cells get usually a very high or very low values such as 9999 or -9999. Before plotting, we should replace these values with nans. Usually I find a cell that is no flow. In this example the cell (1,1) is designated as no-flow and set all values that are equal to that no-flow cell to nan.
#!matlab Head = H(1,1).data; Head( Head == Head(1,1) ) = nan; surf(Head) view(0,90)
This figure is better but not quite right yet. The plot is upside down. There are two ways to fix this. Either provide the coordinates i.e. surf(X,Y,Head)
or use ij axis system. The following shows the second way since I dont have a coordinate system. I also dont like to show the cell edges and most of the time I hide them.
#!matlab surf(Head,'edgecolor','none') axis ij view(28,38)
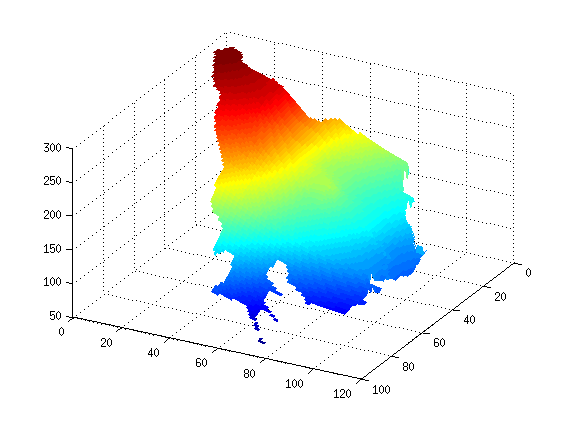
Reading Drawdown output
Reading drowdown is identical to hydraulic head.
#!matlab DDN = readModflowArrays(['Example_files/gvmod.ddn']);
Reading Cell by cell flow file
Reading the cell by cell flow from a user perspecive is not very different than reading hydraulic head
#!matlab CBC = readModflowFlowdata(['Example_files/gvmod.cbb']);
This command will return a struct variable with similar fields as described above.
VERY IMPORTANT NOTE
The readModflowFlowdata
function does not read all types of data. If anyone has files that have properties that this script cannot read and can share the file please contact me at gkourakos@ucdavis.edu
Updated